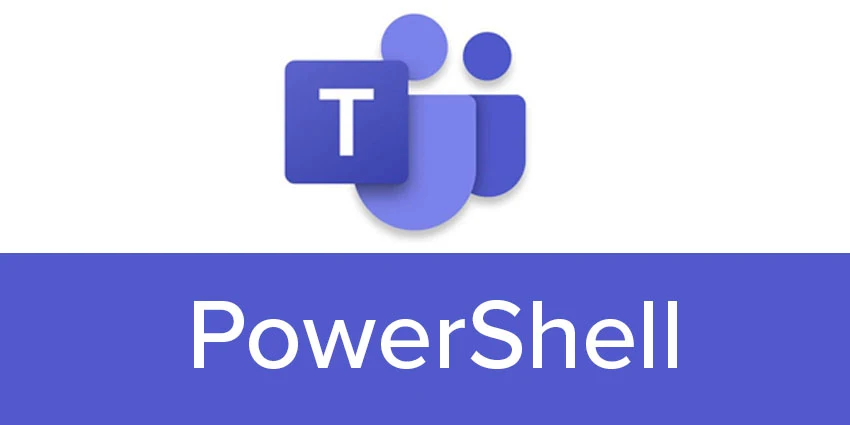
In this article, we'll look at how to send messages to Teams channels from PowerShell using webhook or Microsoft Graph API calls. With PowerShell, you can send or read messages in Teams channels. This can be used in various scenarios for monitoring and notifying events of certain teams not via an email message (Send-MailMessage cmdlet), but directly to a Teams group.
Send a message to Teams via WebHook.
You can send messages to a Microsoft Teams channel using the built-in WebHook connectors. Such a connector is a URI to which a JSON object can be sent using an HTTP POST request.
- Create a separate channel in Teams. You can create a channel using the Microsoft Teams PowerShell module. For example: Get-team -DisplayName sysops| New-TeamChannel -DisplayName “AdminAlerts” -MembershipType Private
- Then open the Teams client (desktop or web) and select the Connectors option from the channel's context menu;
- Add a connector of type Incoming Webhook;
- Specify the name of the connector;
- Copy the connector URL that Azure will generate for you.
To send a simple message to a channel at this URL, run the following PowerShell commands:
$myTeamsWebHook = “https://site.webhook.office.com/webhookb2/123456-12312-@aaaaa-bbbb-cccc/IncomingWebhook/xxxxxxxxxxxxxxxxxxxxx/xxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxxxxxxxxxxx/xxxxx-xxxx-xxxx-xxxx”
Invoke-RestMethod -Method post -ContentType 'Application/Json' -Body '{"text":"Test Teams!"}' -Uri $myTeamsWebHook
Make sure your message appears in the Teams channel. The connector you created is listed as the author of the message.
Teams allows you to send a maximum of 4 requests per second.
You can add additional data to the Teams notification, change the font, color, add additional information from your PowerShell script.
For example, the following script listens for a user lockout event (EventID 4740) on a domain controller with the FSMO PDC role and sends a notification to a Teams channel.
$LockedUser = Search-ADAccount -UsersOnly –LockedOut | Get-ADUser –Properties lockoutTime, emailaddress | Select-Object emailaddress, @{n='lockoutTime';e={[DateTime]::FromFileTime($_.lockoutTime)}} | Sort-Object LockoutTime -Descending | Select-Object -first 1
$myTeamsWebHook = "YOUR-WEBHOOK-URL"
$webhookMessage = [PSCustomObject][Ordered]@{
"@type" = "FF0000"
"@context" = "http://schema.org/extensions"
"summary" = "Locked User: $($LockedUser.SamAccountName) "
"themeColor" = '700015'
"title" = "User Lockout Event"
"text" = "`n
SamAccountName: $($LockedUser.SamAccountName)
Mail: $($LockedUser.EmailAddress)
Timestamp: $($LockedUser.LockoutTime.ToString()) "
}
$webhookJSON = convertto-json $webhookMessage -Depth 50
$webhookCall = @{
"URI" = $myTeamsWebHook
"Method" = 'POST'
"Body" = $webhookJSON
"ContentType" = 'application/json'
}
Invoke-RestMethod @webhookCall
As a result, such an event gets into the Teams channel and an administrator can react to it.
Such a script can be attached to the EventID 4740 event in the Event Viewer. Or you can create a job scheduler and periodically run a PowerShell script.
Send or read a message in Teams using the Microsoft Graph API.
With the Microsoft Graph API, you can not only send, but also read messages from a Teams channel. To do this, you need to register an Azure application, set up permissions (Group.Read.All, ChannelMessage.Send, Chat.ReadWrite and ChatMessage.Send) and get an authentication token.
$ApplicationID = "46692ad-f8a0-123f-8cca-432102de3bcf"
$TenatDomainName = "26216542-465a-407e-a17d-2bb4c3e3313b"
$AccessSecret = "d-8jM3ZUG87du-syZd32k01q.gkssa3mH3v"
$Body = @{
Grant_Type = "client_credentials"
Scope = "https://graph.microsoft.com/.default"
client_Id = $ApplicationID
Client_Secret = $AccessSecret
}
$ConnectGraph = Invoke-RestMethod -Uri https://login.microsoftonline.com/$TenatDomainName/oauth2/v2.0/token -Method POST -Body $Body
$token = $ConnectGraph.access_token
$URLchatmessage="https://graph.microsoft.com/v1.0/teams/$TeamID/channels/$ChannelID/messages"
$BodyJsonTeam = @"
{
"body": {
"content": "Hello World"
}
}
"@
Invoke-RestMethod -Method POST -Uri $URLchatmessage -Body $BodyJsonTeam -Headers -Headers @{Authorization = "Bearer $($token)"}
$TeamID and $ChannelID can be obtained using Get-Team and Get-TeamChannel from the MicrosoftTeams module.
Similarly, using the GET method, you can read messages from a Teams chat.